Page 1 of 2
[SOLVED] Math: Shortest distance from point to line (EDITED)
Posted: Fri Jul 12, 2013 3:59 am
by XHH
I have two line segments. All I have are the coordinates for these line segments. How can I find out if these two line
segments intersect?
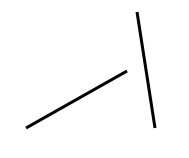
- AlB0e.png (1.99 KiB) Viewed 481 times

Re: [Help] Math-related: Do two line segments intersect?
Posted: Fri Jul 12, 2013 4:10 am
by raidho36
Re: [Help] Math-related: Do two line segments intersect?
Posted: Fri Jul 12, 2013 4:51 am
by XHH
Thank you sir! One more new question though. How do I find the shortest distance from a point to a line?
Re: [Help] Math: Shortest distance from point to line (EDITE
Posted: Fri Jul 12, 2013 5:56 am
by micha
Here is some code that determines the distance of a points from a (infinite) line. If you need the distance of a points from a line segment, it has to be modiefied.
The point is given as (px,py) the lines is given by two points (x1,y1) and (x2,y2)
Code: Select all
function distPointToLine(px,py,x1,y1,x2,y2)
local dx,dy = x1-x2,y1-y2
local length = math.sqrt(dx*dx+dy*dy)
dx,dy = dx/length,dy/length
return math.abs( dy*(px-x1) - dx*(py-y1))
end
Disclaimer: Untested.
This snippet calculates the unit vector pointing along the line (vector of length one). Then this vector is rotated by 90°, which is achieved by swapping x- and y-component and multiplying one with -1, that is (dx,dy) -> (dy,-dx). This is the normal vector for the line. To determine the distance, then the scalar product with any vector from the line to the point p is calculated.
If you leave away the math.abs, then you get a distance with sign. This is useful, if you want to determine if a point crossed the line in one frame (compare the sign of the distance before and after the frame, if the sign is different, the line has been crossed).
Re: [Help] Math: Shortest distance from point to line (EDITE
Posted: Fri Jul 12, 2013 12:11 pm
by XHH
micha wrote:If you need the distance of a points from a line segment, it has to be modiefied.
Can you post that too then?
(thanks)
Re: [Help] Math: Shortest distance from point to line (EDITE
Posted: Fri Jul 12, 2013 1:02 pm
by micha
XHH wrote:Can you post that too then?
(thanks)
Sure. Disclaimer again: This is from the top of my head. Please test.
Code: Select all
function distPointToLine(px,py,x1,y1,x2,y2)
local dx,dy = x2-x1,y2-y1
local length = math.sqrt(dx*dx+dy*dy)
dx,dy = dx/length,dy/length
local posOnLine = dx*(px-x1) + dy*(px-y1)
if posOnline < 0 then
-- first end point is closest
dx,dy = px-x1,py-y1
return math.sqrt(dx*dx+dy*dy)
elseif posOnLine > length then
-- second end point is closest
dx,dy = px-x2,py-y2
return math.sqrt(dx*dx+dy*dy)
else
-- point is closest to some part in the middle of the line
return math.abs( dy*(px-x1) - dx*(py-y1))
end
end
Re: [Help] Math: Shortest distance from point to line (EDITE
Posted: Fri Jul 12, 2013 4:43 pm
by XHH
Didn't completely work. Sometimes it gives me 1.0 as the distance.
Re: [Help] Math: Shortest distance from point to line (EDITE
Posted: Fri Jul 12, 2013 4:45 pm
by raidho36
Re: [Help] Math: Shortest distance from point to line (EDITE
Posted: Fri Jul 12, 2013 5:00 pm
by micha
I found a typo. This one seems to work:
Code: Select all
function distPointToLine(px,py,x1,y1,x2,y2)
local dx,dy = x2-x1,y2-y1
local length = math.sqrt(dx*dx+dy*dy)
dx,dy = dx/length,dy/length
local posOnLine = dx*(px-x1) + dy*(py-y1)
if posOnLine < 0 then
-- first end point is closest
dx,dy = px-x1,py-y1
return math.sqrt(dx*dx+dy*dy)
elseif posOnLine > length then
-- second end point is closest
dx,dy = px-x2,py-y2
return math.sqrt(dx*dx+dy*dy)
else
-- point is closest to some part in the middle of the line
return math.abs( dy*(px-x1) - dx*(py-y1))
end
end
Re: [Help] Math: Shortest distance from point to line (EDITE
Posted: Fri Jul 12, 2013 5:13 pm
by Ref
Saw the typo and after correction seems to work for me.
Question:
Easy to find closest-point-on-line but pretty mess to get closest-point-on-line-segment.
Do you have a 'neat' way of doing this?