I'm currently prototyping a game out, I have a bunch of tiles in a quad and I'm drawing them based on a grid. The problem I am having, after scaling upwards (using gamera, not sure if that's relevant or not), I am seeing artefacts when moving. I've taken some screenshots:
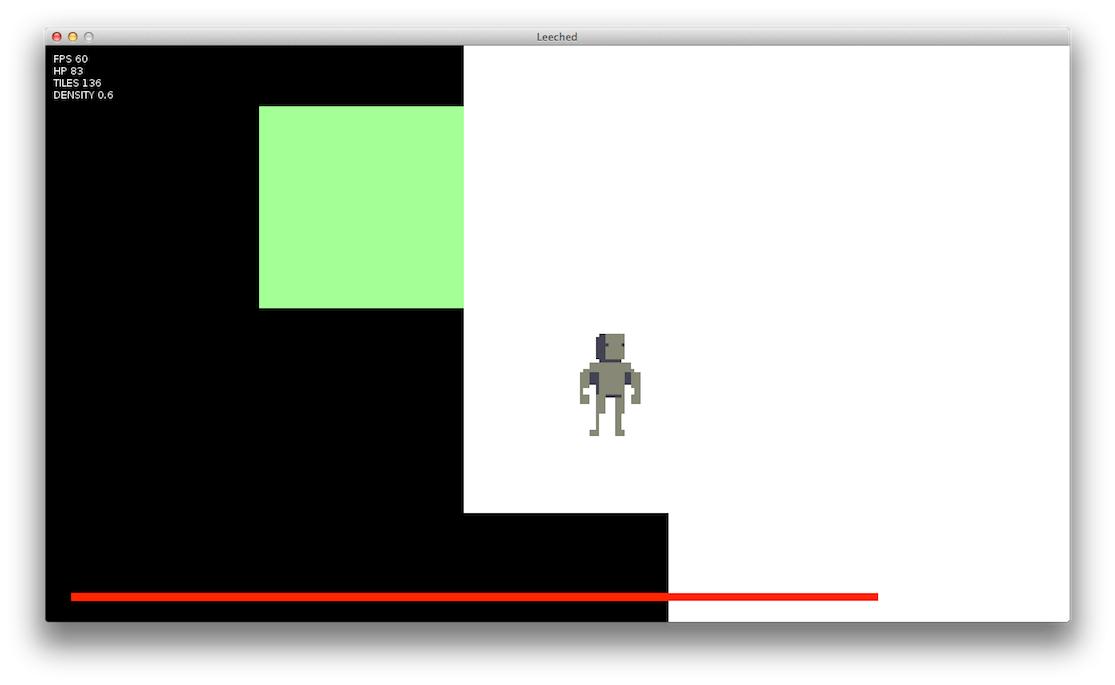
This one is fine
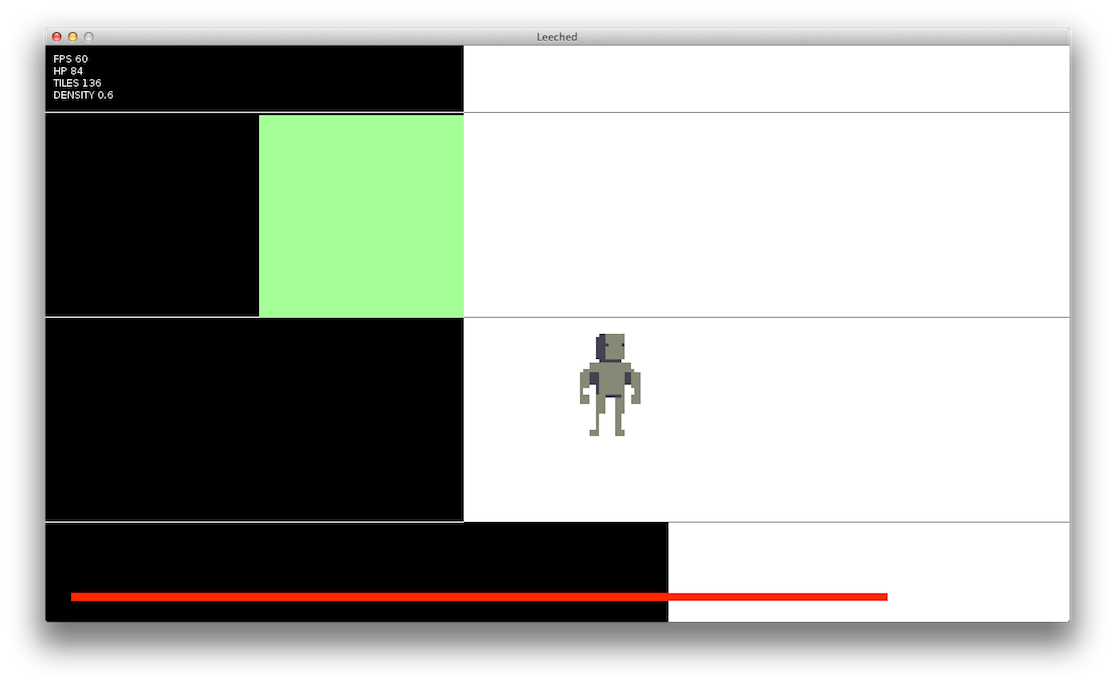
Notice the white lines. They sort of flicker, not always visible.
I have love.graphics.setDefaultFilter('nearest', 'nearest') set.
The tileset I'm using:
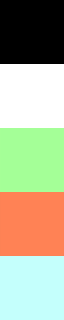
Think I am loading the quads in a normal fashion:
Code: Select all
Quads = {
love.graphics.newQuad(0, 0, tileW, tileH, tilesetW, tilesetH),
love.graphics.newQuad(0, 64, tileW, tileH, tilesetW, tilesetH),
love.graphics.newQuad(0, 128, tileW, tileH, tilesetW, tilesetH),
love.graphics.newQuad(0, 192, tileW, tileH, tilesetW, tilesetH),
love.graphics.newQuad(0, 256, tileW, tileH, tilesetW, tilesetH)
}
Edit: Probably worth noting that I have
Code: Select all
for rowIndex=1, #dungeon do
local row = dungeon[rowIndex]
for columnIndex=1, #row do
local number = row[columnIndex]
love.graphics.draw(tileSet, Quads[number], (columnIndex-1)*tileW, (rowIndex-1)*tileH)
end
end