If using 3D engine like groverburger's and i want to ask if it is possible to draw a static image onto the background before drawing the 3D stuff. That means, i will see the picture as "sky" for example and then the flying airplanes as 3D stuff on the sky.
This way i could make parallax background with 3D ships. Or any other cool effects without the need for models.
I do not want to create any skydome or skyboxes for this. Just a plain background texture for depth buffer i quess?
My solution would be work through shader. I quess that i need to send the texture pointer into the shader and from there put it into the depth buffer into the distance. But my knowledge about shader working is not yet strong.
Any help with this please?
Just some notes:
- gl_fragColor.z for distance in the background
- gl_fragColor.x and y will be calculated automatically
- propably i need at least a quad vertex model mapped to texture UV that will be calculated based on the screen width and height
background in 3D [SOLVED]
background in 3D [SOLVED]
Last edited by Rigachupe on Sat Jun 10, 2023 3:42 pm, edited 1 time in total.
Re: background in 3D
You could use multiple canvases: a background canvas where you draw your parallax and a depth-enabled canvas with your 3d scene. You can then draw your canvases in the correct order on the screen to achieve this effect (i've used the same trick when i've played around with groverburger's 3d engine).
Re: background in 3D
If you don't want it to move in relation to the camera you can just draw the image onto the screen using the normal 2D API before doing any of the g3d drawing.
Re: background in 3D
I tried it but it did not work.
1. i set camera 2D perspective, then i draw a image to screen (depth buffer should not be affected)
2. i set camera 3D perspective, then i draw a model to screen (depth buffer affacted)
3. result is i see only the 2D image and the 3D model is behind it
Re: background in 3D
This could work in my opinion. But i have no idea how to work with canvases in g3d. :-( Any example perhaps?Tabaqui wrote: ↑Wed Feb 15, 2023 8:18 am You could use multiple canvases: a background canvas where you draw your parallax and a depth-enabled canvas with your 3d scene. You can then draw your canvases in the correct order on the screen to achieve this effect (i've used the same trick when i've played around with groverburger's 3d engine).
Re: background in 3D
What I actually meant was to just do something like this:Rigachupe wrote: ↑Thu Feb 16, 2023 6:00 am I tried it but it did not work.
1. i set camera 2D perspective, then i draw a image to screen (depth buffer should not be affected)
2. i set camera 3D perspective, then i draw a model to screen (depth buffer affacted)
3. result is i see only the 2D image and the 3D model is behind it
Code: Select all
local sx=love.graphics.getWidth()/bgImg:getWidth()
local sy=love.graphics.getHeight()/bgImg:getHeight()
love.graphics.draw(bgImg,0,0,0,sx,sy)
...
g3d stuff
...
Edit: FYI setting the camera to an orthographic projection doesn't stop it from updating the the depth buffer. You need to configure the render canvas to ignore depth buffer writes for this to happen, see https://love2d.org/wiki/love.graphics.setDepthMode. I suspect g3d is doing this under the hood so it may overwrite anything you do manually, not sure though.
Edit2: setDepthMode isn't the right thing for stopping depth buffer writes. I think the first thing I said was correct though.

Re: background in 3D
I'm the same as marclurr's.
You can composite the screen by ordering the drawing commands from background elements to foreground elements, such that you draw the image first, then 3D elements etc.
This is called the Painter's Algorithm.
Each frame before calling love.draw(), Löve clears the screen for you (this is done by the default code assigned to the love.run() function).
After this, inside your love.draw(), the order in which you draw things will be the order that they appear on screen, layered onto each other.
If you want to draw 2D graphics, then 3D, then 2D again, then some more 3D etc that's perfectly doable. You just need to make sure that the viewport and projection settings are properly set up for this new thing that you're about to draw, whether 2D or 3D.
PS marclurr you were right the first time, love.graphics.setDepthMode() is both used to set the depth-test function as well as the write flag -- it's the second parameter.
You can composite the screen by ordering the drawing commands from background elements to foreground elements, such that you draw the image first, then 3D elements etc.
This is called the Painter's Algorithm.
Each frame before calling love.draw(), Löve clears the screen for you (this is done by the default code assigned to the love.run() function).
After this, inside your love.draw(), the order in which you draw things will be the order that they appear on screen, layered onto each other.
If you want to draw 2D graphics, then 3D, then 2D again, then some more 3D etc that's perfectly doable. You just need to make sure that the viewport and projection settings are properly set up for this new thing that you're about to draw, whether 2D or 3D.
PS marclurr you were right the first time, love.graphics.setDepthMode() is both used to set the depth-test function as well as the write flag -- it's the second parameter.
Re: background in 3D
...continued.
The smart ordering of drawing commands plus the change in viewport & model/view/projection settings lets you do cool compositing things, like in this screenshot for Radiata Stories (PS2), where it draws the game camera, then an animated 3D portrait, then the 2D text, all during a dialog scene:
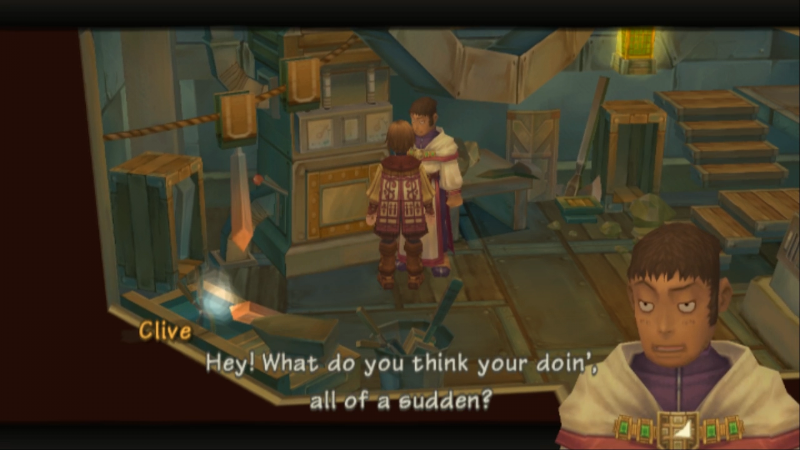
Or the little animated portrait at the bottom of the screen in RTS games, like Warcraft 3. I think in this case it's, drawing the game camera, then the 3D portrait, then the 2D UI, with a hole where the portrait is:

The smart ordering of drawing commands plus the change in viewport & model/view/projection settings lets you do cool compositing things, like in this screenshot for Radiata Stories (PS2), where it draws the game camera, then an animated 3D portrait, then the 2D text, all during a dialog scene:
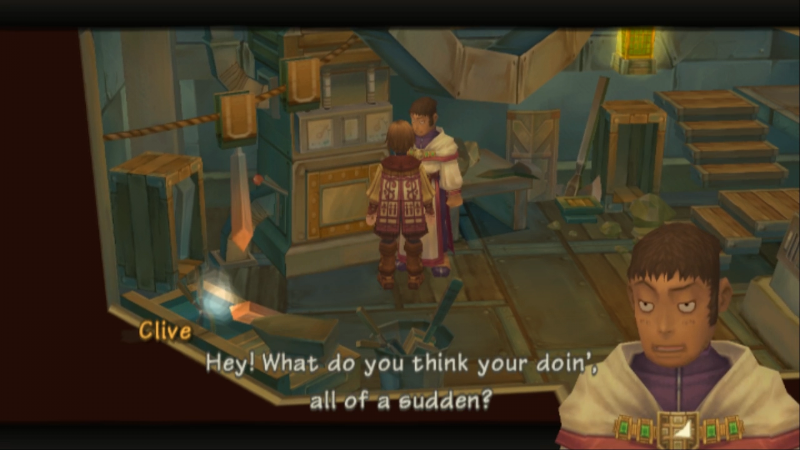
Or the little animated portrait at the bottom of the screen in RTS games, like Warcraft 3. I think in this case it's, drawing the game camera, then the 3D portrait, then the 2D UI, with a hole where the portrait is:

Re: background in 3D
Sure, this is how i would do it:
Code: Select all
-- Requires
-- ...
function love.load()
local w = ...
local h = ...
canvas2d = love.graphics.newCanvas(w, h)
canvas3d = love.graphics.newCanvas(w, h)
end
function love.draw()
-- Draw 2d and 3d stuff on your canvases first
love.graphics.setCanvas(canvas2d)
love.graphics.clear()
draw2dStuff()
love.graphics.setCanvas({canvas3d, depth = true})
love.graphics.clear()
draw3dStuff()
-- Draw your canvases on screen
love.graphics.setCanvas()
love.graphics.draw(canvas2d, 0, 0)
love.graphics.draw(canvas3d, 0, 0)
end
function draw2dStuff()
-- Here you can draw textures/lines/polygons etc... using love2d API
-- ...
end
function draw3dStuff()
-- And here you can draw your models using g3d
-- ...
end
Re: background in 3D
Thx, got it now for testing.
Who is online
Users browsing this forum: Google [Bot] and 3 guests