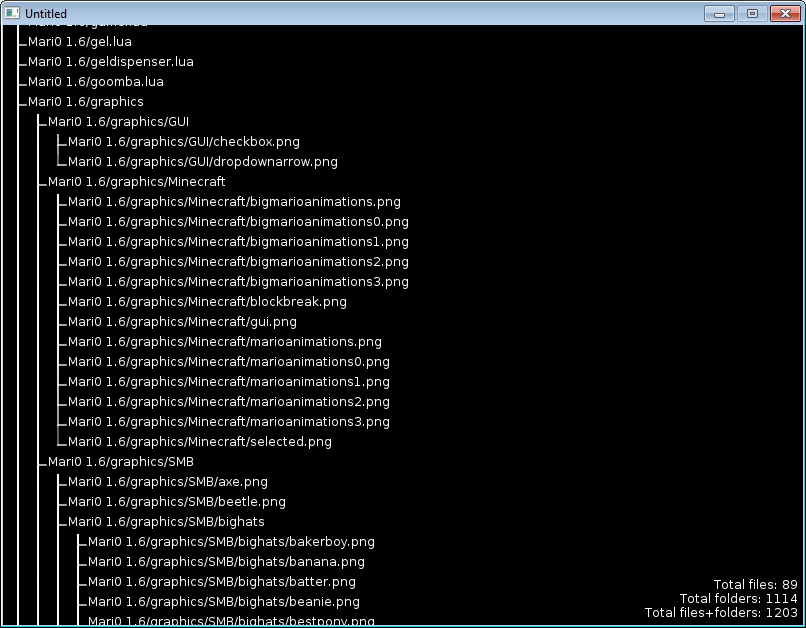
Code: Select all
function love.load()
font = love.graphics.newFont(12)
fontbig = love.graphics.newFont(24)
love.graphics.setFont(fontbig)
totalfiles = 0
totalfolders = 0
t = rE("")
love.graphics.setFont(font)
offset = 0
nameToPrint = "" --replace this with "[FOLDERNAME]/" so you can also show the folder where main.lua is
end
function love.draw()
rP(t, 5, 5, 20, 20, offset, font:getHeight())
local txt = "Total files: " .. totalfiles
love.graphics.print(txt, 800-5-font:getWidth(txt), 600-5-font:getHeight()*3)
txt = "Total folders: " .. totalfolders
love.graphics.print(txt, 800-5-font:getWidth(txt), 600-5-font:getHeight()*2)
txt = "Total files+folders: " .. totalfiles+totalfolders
love.graphics.print(txt, 800-5-font:getWidth(txt), 600-5-font:getHeight())
end
function rE(f)
loading(f)
local t = {}
local files = love.filesystem.enumerate(f)
local folder = f
if folder ~= "" then folder = folder .. "/" end
for i, v in pairs(files) do
if love.filesystem.isFile(folder .. v) then
totalfolders = totalfolders + 1
table.insert(t, folder .. v)
else
totalfiles = totalfiles + 1
table.insert(t, rE(folder .. v))
end
end
return {f, t}
end
function loading(f)
love.graphics.clear()
love.graphics.print("Loading:", 400-fontbig:getWidth("Loading:")/2, 300-fontbig:getHeight())
love.graphics.print(f, 400-fontbig:getWidth(f)/2, 300)
love.graphics.present()
end
function rP(t, x, y, tx, ty, off, fh, hasline)
local hasline = hasline or false
local y = y
local first = true
local lasty = y
for i, v in pairs(t[2]) do
if type(v) == "table" then
if y-off+fh >= 0 then
love.graphics.print(nameToPrint .. v[1], x, y-off)
end
if hasline then
local ny = lasty-off
if first then ny = y-off end
if y+ty/2-off > 0 then
if ny < 600 then
love.graphics.line(x-tx/2, ny, x-tx/2, y+ty/2-off)
else
return y
end
end
if y+ty/2-off < 600 and y+ty/2-off > 0 then
love.graphics.line(x-tx/2, y+ty/2-off, x-2, y+ty/2-off)
end
end
y = rP(v, x+tx, y+ty, tx, ty, off, fh, true)
y = y - ty
else
if y-off+fh >= 0 then
love.graphics.print(nameToPrint .. v, x, y-off)
end
if hasline then
local ny = lasty-off
if first then ny = y-off end
if y+ty/2-off > 0 then
if ny < 600 then
love.graphics.line(x-tx/2, ny, x-tx/2, y+ty/2-off)
else
return y
end
end
if y+ty/2-off < 600 and y+ty/2-off > 0 then
love.graphics.line(x-tx/2, y+ty/2-off, x-2, y+ty/2-off)
end
end
end
y = y + ty
first = false
end
return y
end
function love.mousepressed(x, y, button)
local v = 15
if love.keyboard.isDown("lshift") or love.keyboard.isDown("lctrl") then v = 30 end
if button == "wu" then
offset = math.max(0, offset - 15)
elseif button == "wd" then
offset = math.min((totalfiles+totalfolders)*20-600, offset + 15)
end
end
