Any global modules get their class-table-object imported in camelcase, just like the in the actual file. (The modules all extend rsi's class module as 'Obect') However local modules all come in as lowercase just like the filename.
This is what I mean:

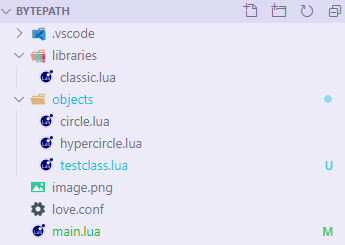
Any idea why this is happening? Ideally, I'd like them to come in to match the class/table style in camelcase. But mostly, I'd like to understand what is going on here.
**main.lua**
Code: Select all
--! file: main.lua
Object = require "libraries.classic"
local object_files = {}
local c1, h1
function string:split( inSplitPattern )
local outResults = {}
local theStart = 1
local theSplitStart, theSplitEnd = string.find( self, inSplitPattern, theStart )
while theSplitStart do
table.insert( outResults, string.sub( self, theStart, theSplitStart-1 ) )
theStart = theSplitEnd + 1
theSplitStart, theSplitEnd = string.find( self, inSplitPattern, theStart )
end
table.insert( outResults, string.sub( self, theStart ) )
return outResults
end
function love.keypressed(key, u)
--Debug
if key == "rctrl" then
debug.debug()
end
end
function recursiveEnumerate(folder, file_list)
local filesTable = love.filesystem.getDirectoryItems(folder)
for i,v in ipairs(filesTable) do
local file = folder .. '/' .. v
if love.filesystem.getInfo(file, 'file') then
table.insert(file_list, file)
elseif love.filesystem.getInfo(file, 'directory') then
recursiveEnumerate(file, file_list)
end
end
end
function requireFiles(files)
for _,filepath in ipairs(files) do
local filepath = filepath:sub(1, -5)
-- using a split function because lua doesn't have one built-in
local parts = filepath:split("/")
local class = parts[#parts]
_G[class] = require(filepath)
end
end
function love.load()
recursiveEnumerate('objects', object_files)
requireFiles(object_files)
image = love.graphics.newImage('image.png')
c1 = Circle(400, 300, 50)
hc1 = HyperCircle(400, 300, 50, 50, 50)
end
function love.update(dt)
c1:update(dt)
hc1:update(dt)
end
function love.draw()
for i,v in ipairs(object_files) do
love.graphics.print(object_files[i], 20, 20 + (i * 10))
end
c1:draw()
hc1:draw()
end
Code: Select all
Circle = Object:extend()
function Circle:new(x, y, radius)
self.x = x
self.y = y
self.radius = radius
self.creation_time = love.timer.getTime()
end
function Circle:update(dt)
end
function Circle:draw()
love.graphics.circle('fill', self.x, self.y, self.radius)
end
Code: Select all
HyperCircle = Circle:extend()
local distance
local widthy
function HyperCircle:new(x, y, radius, outer_radius_distance, line_width)
HyperCircle.super.new(self, x, y)
self.radius = radius
self.outer_radius = self.radius + outer_radius_distance
self.line_width = line_width
distance = self.outer_radius
widthy = self.line_width
end
function HyperCircle:update(dt)
self.outer_radius = love.math.random(self.radius, distance + 200)
self.line_width = love.math.random(1, widthy)
end
function HyperCircle:draw()
love.graphics.setLineWidth(self.line_width)
love.graphics.circle('line', self.x, self.y, self.outer_radius)
end
Code: Select all
local TestClass = Object:extend()
function TestClass:new()
end
function TestClass:update()
end
function TestClass:draw()
end
return TestClass