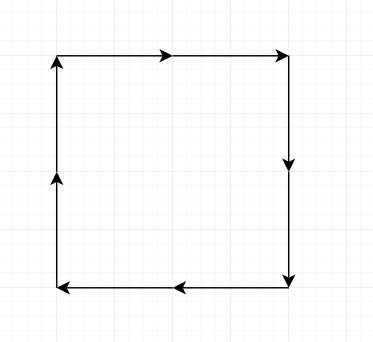
Love2D requires this to be convex (Box2d):
Creates a new PolygonShape.
This shape can have 8 vertices at most, and must form a convex shape.
As the game proceeds and the player is rewarded, the shape grows by moving one 'point' "outwards", but it must do so without breaking the "convex" rule:
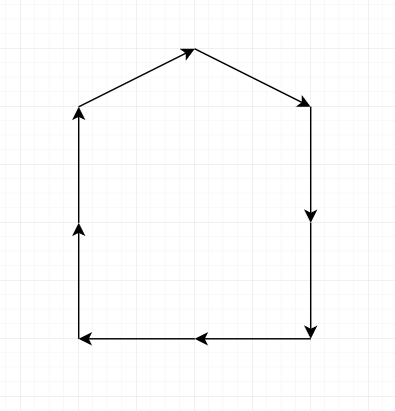
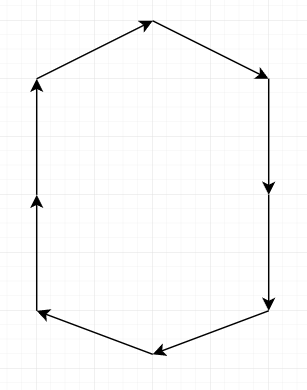
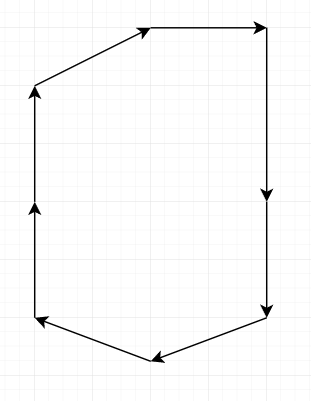
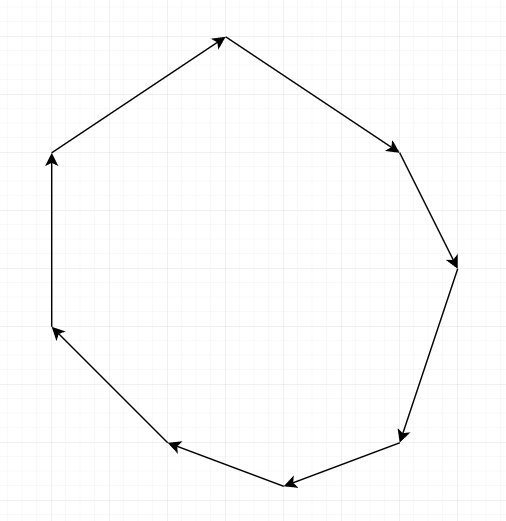
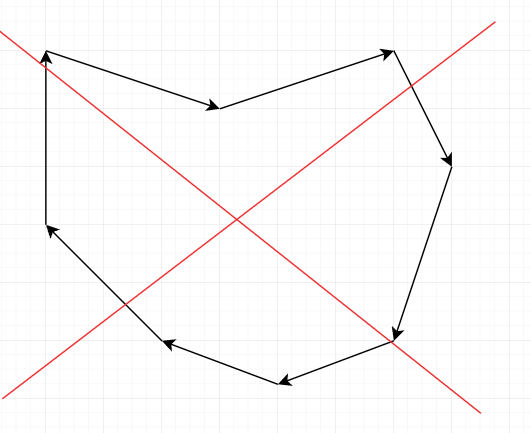
The shape can get quite large and quite complex as the player keeps winning and getting rewarded. Over time, the size of the shape starts to work against the player and that is one of the mechanics of the game.
Here is the challenge:
Assuming a 'point' is the end of one segment and the start of the next segment:
- Starting with the square, each time the player receives a reward, two segments (or one 'point') are moved so that the shape gets larger, i.e. the inside area increases.
- The shape must always be convex
- The 8 'points' of the convex can move according to whatever rules you invent (i.e. along axis or random or whatever)
- Any point can be moved according to described rules
- Assume moving a 'point' is by an arbitrary distance (unless it matters) but for simplicity, assume whole numbers of pixels
- You can assume that moving the end of one segment means moving the start of the segment it joins
- Bonus points if the shape formed is irregular i.e. not a perfect square or rectangle.