I mean you know how it ripples. Not quite a sine wave pattern but more an abnormal pattern of jaggy offsets moving in a smooth wave pattern. But I just don't know how I would shift a row of pixels or something.
I mean right now I just have this pretty looking thing:
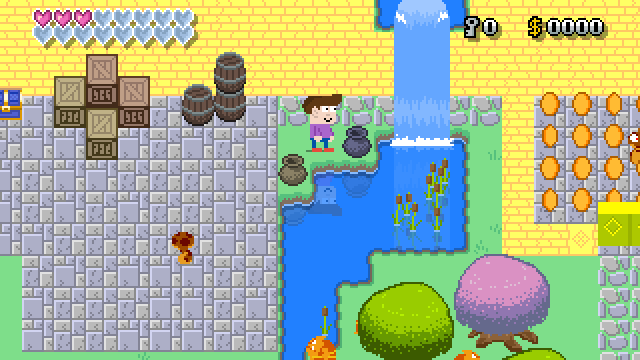
It's not that big a deal. I'm drawing each reflectable entity onto a separate field that gets placed below the world and the water is a transparent blue texture. But I'd like to do something cool with the reflection layer just to make it look neater.